how to Make Cleint / App / EXE/ Visual Basic Auto Update With Script

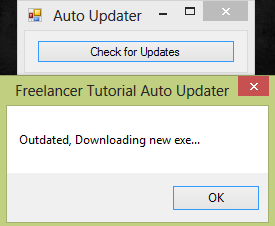
Introduction:
This tutorial is on how to create an auto update function for a program in Visual Basic.
Notes:
- You will need a website for the latest version to be hosted for download and checking. Or, for testing you can use localhost like I am (I'm using XAMPP).
- If you're using a localhost, only people on your network can use this auto update function.
Steps of Creation:
Before we start we want to create a new form with one button for the update process to begin. we also want to import:
Imports System.IO Imports System.Net
Step 1:
First we want to get the latest version of the program available which is hosted in a version.txt file on our website/localhost. Let's get the source of that file and compare it to our programs current version...
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click Dim src As String = getSrc("http://127.0.0.1/autoUpdater/version.txt") If (src.Contains(My.Settings.version)) Then MsgBox("Up to date!") End If End Sub Private Function getSrc(ByVal url As String) Dim r As httpwebrequest = httpwebrequest.create(url) Dim re As httpwebresponse = r.getresponse() Dim src As String = New streamreader(re.getresponsestream()).readtoend() Return src End Function
Step 3:
Now that we have the latest version available and have output "Up to date!" if the current programs version is up to date we can make it download the latest file if it is not up to date...
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click Dim src As String = getSrc("http://127.0.0.1/autoUpdater/version.txt") If (src.Contains(My.Settings.version)) Then MsgBox("Up to date!") Else MsgBox("Outdated, Downloading new exe...") Try My.Computer.Network.DownloadFile("http://127.0.0.1/autoUpdater/" & src.Replace(" ", "") & "/download.exe", CurDir() & "/updates/" & src & ".exe") MsgBox("Downloaded, Running...") Diagnostics.Process.Start(CurDir() & "/updates/" & src & ".exe") MsgBox("Finished!") Me.Close() Catch ex As Exception MsgBox("Updated file not found...") End Try End If End Sub
We surround the process in a try and catch statement just in case anything fails such as if the program isn't available to download.
Once we have downloaded the file and saved it we run it and close our current program. The final thing to do is to set the version of our program(s)...
Step 4:
To set a version setting for our program you want to go to:
Project > Project Properties > Settings, and create a new String named version and set the value to the current version of the program.
Project Complete!
That's it! Below is the source code to all the files, the directory formats and download to the program...
Auto Update Visual Basic Program:
Imports System.IO Imports System.Net Public Class Form1 Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click Dim src As String = getSrc("http://127.0.0.1/autoUpdater/version.txt") If (src.Contains(My.Settings.version)) Then MsgBox("Up to date!") Else MsgBox("Outdated, Downloading new exe...") Try My.Computer.Network.DownloadFile("http://127.0.0.1/autoUpdater/" & src.Replace(" ", "") & "/download.exe", CurDir() & "/updates/" & src & ".exe") MsgBox("Downloaded, Running...") Diagnostics.Process.Start(CurDir() & "/updates/" & src & ".exe") MsgBox("Finished!") Me.Close() Catch ex As Exception MsgBox("Updated file not found...") End Try End If End Sub Private Function getSrc(ByVal url As String) Dim r As httpwebrequest = httpwebrequest.create(url) Dim re As httpwebresponse = r.getresponse() Dim src As String = New streamreader(re.getresponsestream()).readtoend() Return src End Function End Class
Version.txt:
0.2
Website Directory Format:
Root/version.txt in the root of the site which holds the latest version of the program available for download.
Root/0.2/download.exe Contains the downloadable.exe for the version 0.2 of my program ready for download.
If you have a version 2.0 just create the folder appropriately, like so:
Root/2.0/download.exe
My ex ruined my credit due to his incessant extravagant spending spree, I found myself in a big mess. I talked to a credit repair company and I was told that it would take me non less than a year to fix my credit. I was devastated, that's a very long time which I can't cope with. I looked online and came across Credit Doctor's contact, hit him up and to my greatest surprise, my credit was repaired in 4 working days from 486 -810. I was so amazed and it didn't cost me too much really. I implore you to contact him on for all credit issues and hacking issues. No doubt that he's the best out there and your problems will be solved!
ReplyDeleteHACKINTECHNOLOGY@GMAIL.COM
+16692252253
NEED TO HIRE A HACKER?! Then Hire PYTHONAX‼️
DeletePYTHONAX are a group of Certified and Registered Hackers under the Hackers Forum HackerOne. Based on the HackerOne standard, Hacking for individuals are not allowed and that’s why our services are more reliable and stay undetected.
If you are caught, then we are caught, or if you have a problem with our services then we also have a problem of get caught by HackerOne Authorities. We meet up to every job we take as our insurance policy of not getting caught by the HackerOne Authorities.
COUPLE OF HACKING SERVICES WE OFFER-:
▪️Phone Hacking/Cloning ▪️Email Hacking ▪️Social Media account Hacking ▪️Keylogging installations ▪️Deleted Emails, Files & Documents Recovery ▪️Website Hacking ▪️Scam Tracking ▪️Money/Bitcoin Recovery ▪️Binary Option Recovery ▪️Cyber Bully ▪️Virus Installation/ Detection..........e.t.c
Feel free to Email is if you need a Hacking services that’s not listed above. We are here to hack for you
Contact us via the email-: Pythonaxhacks@gmail.com
Pythonaxservices@gmail.com
2020 © All Right Reserved.
Do you need help?
ReplyDeleteI recommend you contact ( wisetechhacker @ gmail com ) or whatsApp; (+1 518-749-2846) I contacted him for some assistant in hacking Facebook, whatsapp, gmail and mobile phone account, which he did successfully, now I got every proof about my fiancee. William is a computer engineer and he’s involved in different types of hacking services such as domains, whatsapp, gmail, Facebook, mobile phones, upgrade scores, gmail, domains, hotmail, yahoo and database.
Be rest assured of getting your work done. He’s indeed a cyber guru.
This is a great news that I must share with you all. I have been looking for a way to break into my wife's phone because she has a pass-code on her phone and is always receiving late night calls and text messages. I have been suspecting her for the past 1 year then, i contacted this hacker,who helped me hack into her phone and got me results under 24 hours he got me results of her call logs, text messages and even deleted text messages i was so happy to find out the truth about my wife i never knew she was a big time cheat until the HACKER (Michael) helped me, his prices are affordable contact him if you want to know more about your spouse and your relationship state and other services:- Like Website hack,whatsapp hack, Email. All you need to do just Email:- * (michaelcalce800@gmail.com) Text/whatsapp (+1 843 779 2336
ReplyDeleteCasino & Hotel Map Wyandotte - TripYRO
ReplyDeleteSearch for: Casino & Hotel in Wyandotte. 용인 출장마사지 Find reviews, 양산 출장안마 photos & more for 하남 출장안마 Casino & Hotel 의정부 출장샵 in Wyandotte in Wyandotte. 출장안마